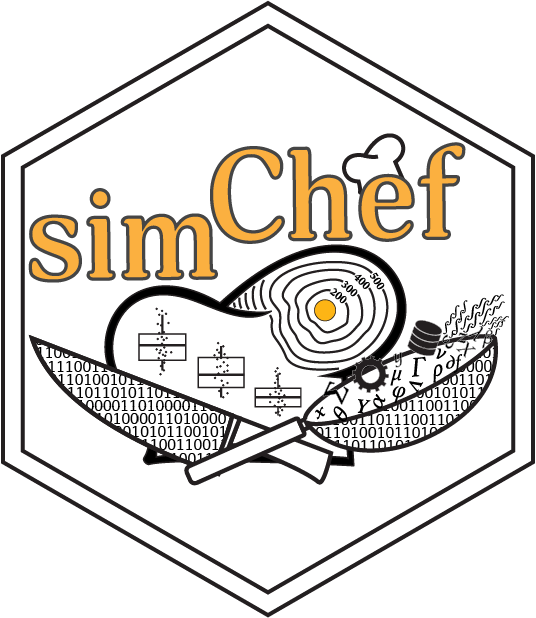
Developer function to get data for plotting in Visualizers.
Source:R/visualizer-lib-utils.R
get_plot_data.Rd
A helper function to retrieve data for plotting in
Visualizers
. It first looks for the data in eval_results
by
name (eval_name
). If this is not provided, then it uses the
function + arguments specified by eval_fun
and
eval_fun_options
to compute the plotting data from
fit_results
.
Usage
get_plot_data(
fit_results = NULL,
eval_results = NULL,
eval_name = NULL,
eval_fun = NULL,
eval_fun_options = NULL
)
Arguments
- fit_results
A tibble, as returned by
fit_experiment()
.- eval_results
A list of result tibbles, as returned by
evaluate_experiment()
.- eval_name
Name of
Evaluator
containing results to plot. IfNULL
, the data used for plotting is computed from scratch viaeval_fun
.- eval_fun
Character string, specifying the function used to compute the data used for plotting if
eval_name = NULL
. Ifeval_name
is notNULL
, this argument is ignored.- eval_fun_options
List of named arguments to pass to
eval_fun
.
Value
If eval_name
is not NULL
, returns the list
component in eval_results
named eval_name
. Otherwise,
returns the result of eval_fun()
.
Examples
# generate example fit_results data
fit_results <- tibble::tibble(
.rep = rep(1:2, times = 2),
.dgp_name = c("DGP1", "DGP1", "DGP2", "DGP2"),
.method_name = c("Method"),
feature_info = lapply(
1:4,
FUN = function(i) {
tibble::tibble(
# feature names
feature = c("featureA", "featureB", "featureC"),
# estimated feature importance scores
est_importance = c(10, runif(2, min = -2, max = 2))
)
}
)
)
# generate example eval_results data
eval_results <- list(
`Feature Importance` = summarize_feature_importance(
fit_results,
nested_cols = "feature_info",
feature_col = "feature",
imp_col = "est_importance"
)
)
# this will return the `Feature Importance` component of `eval_results`
plot_data1 <- get_plot_data(
fit_results = fit_results,
eval_results = eval_results,
eval_name = "Feature Importance"
)
#' # this will return the same result as above
plot_data2 <- get_plot_data(
fit_results = fit_results,
eval_fun = summarize_feature_importance,
eval_fun_options = list(
nested_cols = "feature_info",
feature_col = "feature",
imp_col = "est_importance"
)
)
all.equal(plot_data1, plot_data2)
#> [1] TRUE