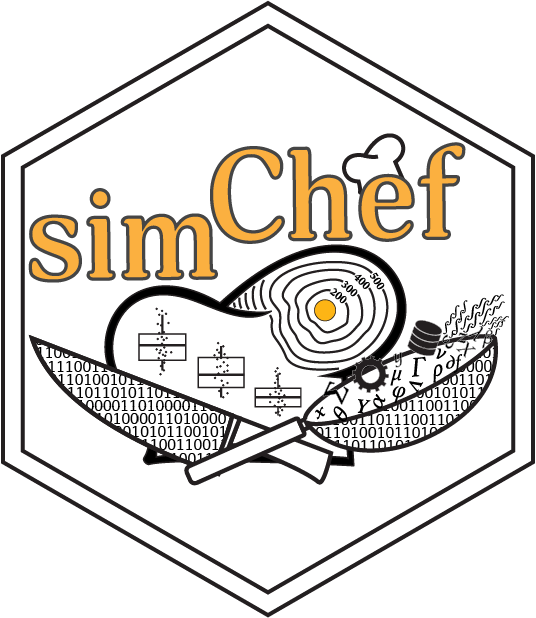
Helper functions for removing components of an Experiment
.
Source: R/experiment-helpers.R
remove_funs.Rd
Helper functions for removing DGPs
, Methods
,
Evaluators
, and Visualizers
already added to an
Experiment
.
Usage
remove_dgp(experiment, name = NULL, ...)
remove_method(experiment, name = NULL, ...)
remove_evaluator(experiment, name = NULL, ...)
remove_visualizer(experiment, name = NULL, ...)
Arguments
- experiment
An
Experiment
object.- name
A name to identify the object to be removed. If
NULL
(default), remove all objects of that class from the experiment. For example,remove_dgp()
will remove all DGPs from the experiment.- ...
Not used.
Examples
## create toy DGPs, Methods, Evaluators, and Visualizers
# generate data from normal distribution with n samples
normal_dgp <- create_dgp(
.dgp_fun = function(n) rnorm(n), .name = "Normal DGP", n = 100
)
# generate data from binomial distribution with n samples
bernoulli_dgp <- create_dgp(
.dgp_fun = function(n) rbinom(n, 1, 0.5), .name = "Bernoulli DGP", n = 100
)
# compute mean of data
mean_method <- create_method(
.method_fun = function(x) list(mean = mean(x)), .name = "Mean(x)"
)
# evaluate SD of mean(x) across simulation replicates
sd_mean_eval <- create_evaluator(
.eval_fun = function(fit_results, vary_params = NULL) {
group_vars <- c(".dgp_name", ".method_name", vary_params)
fit_results %>%
dplyr::group_by(dplyr::across(tidyselect::all_of(group_vars))) %>%
dplyr::summarise(sd = sd(mean), .groups = "keep")
},
.name = "SD of Mean(x)"
)
# plot SD of mean(x) across simulation replicates
sd_mean_plot <- create_visualizer(
.viz_fun = function(fit_results, eval_results, vary_params = NULL,
eval_name = "SD of Mean(x)") {
if (!is.null(vary_params)) {
add_aes <- ggplot2::aes(
x = .data[[unique(vary_params)]], y = sd, color = .dgp_name
)
} else {
add_aes <- ggplot2::aes(x = .dgp_name, y = sd)
}
plt <- ggplot2::ggplot(eval_results[[eval_name]]) +
add_aes +
ggplot2::geom_point()
if (!is.null(vary_params)) {
plt <- plt + ggplot2::geom_line()
}
return(plt)
},
.name = "SD of Mean(x) Plot"
)
# initialize experiment with toy DGPs, Methods, Evaluators, and Visualizers
# using piping %>% and add_* functions
experiment <- create_experiment(name = "Experiment Name") %>%
add_dgp(normal_dgp) %>%
add_dgp(bernoulli_dgp) %>%
add_method(mean_method) %>%
add_evaluator(sd_mean_eval) %>%
add_visualizer(sd_mean_plot)
print(experiment)
#> Experiment Name: Experiment Name
#> Saved results at: results/Experiment Name
#> DGPs: Normal DGP, Bernoulli DGP
#> Methods: Mean(x)
#> Evaluators: SD of Mean(x)
#> Visualizers: SD of Mean(x) Plot
#> Vary Across: None
# example usage of removing DGPs, Methods, Evaluators, and Visualizers
experiment <- experiment %>%
remove_dgp("Normal DGP") %>%
remove_dgp("Bernoulli DGP") %>%
remove_method("Mean(x)") %>%
remove_evaluator("SD of Mean(x)") %>%
remove_visualizer("SD of Mean(x) Plot")
print(experiment)
#> Experiment Name: Experiment Name
#> Saved results at: results/Experiment Name
#> DGPs:
#> Methods:
#> Evaluators:
#> Visualizers:
#> Vary Across: None